1. Introduction
Simples consists of a collection of Python modules and classes for accessing and manipulating B2000++ databases. In addition, it provides a FE mesh modeling module and means for generating B2000++ input files from this module.
In its present version, Simples is closely linked to the B2000++ FE analysis system. The Simples modules extract, manipulate, and view Finite Element based calculations performed with the B2000++ FE analysis program. Simples is programmed with the Python programming language, as is the API.
Simples is intended to replace the data extraction functions of baspl++, since baspl++ does not work with Python 3.
Example 1: Extract time step versus displacement function values of a viscoelastic test specimen loaded with a constant pulling force applied a time \(t=0\) and kept constant for the duration of the time integration.
Start by launching a Python shell and by importing the Simples
modules and opening the model database
of the
B2000 model visco
:
import simples as si
model = si.Model('visco')
Extract the time (step) values (method
get_steps()
):
x = model.get_steps()
Extract the values of the displacement: (method
get_dof_value_of_steps()
):
y = model.get_dof_value_of_steps('displacements', 3, 1)
Generate the diagram with the Graph2
class
and save it in the file output.png
:
g = si.Graph2()
g.add_line(x, y)
g.save('output.svg')
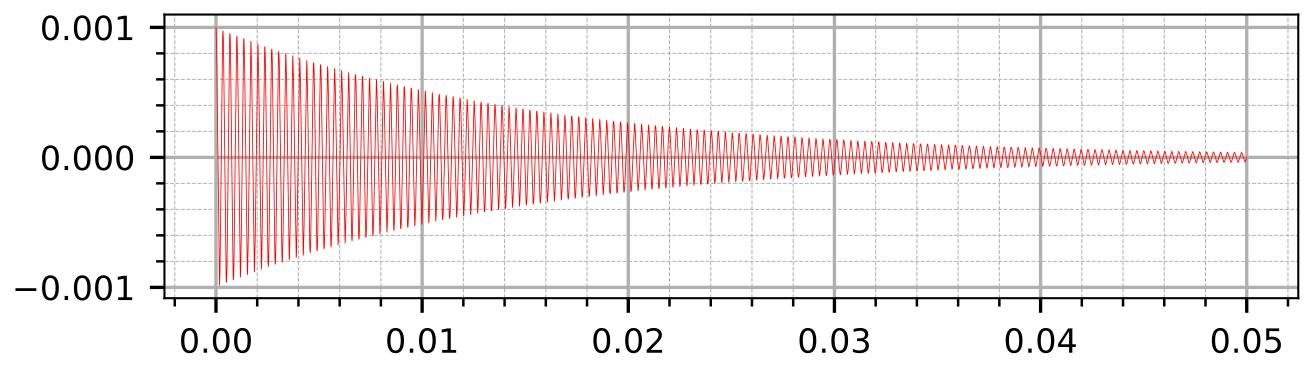
File output.png
Example 2: Extract the load versus end-shortening function values
of a post-buckling analysis of a compressed cylindrical panel and
generate the diagram in the file output.png
:
import simples as si
# Open model 'panel'
m = si.Model('panel')
# Get load steps and sum of reaction forces of each load step
x = m.get_steps()
y = m.get_reaction_force_of_steps(what='ebc', eid=2)
# Plot curve: Create a graph, add the curve, and create figure
g=si.Graph2()
line=g.add_line(x, y)
g.axes_label_x = 'Stroke [mm]'
g.axes_label_y = 'Compression force [N]'
g.save('output.png')
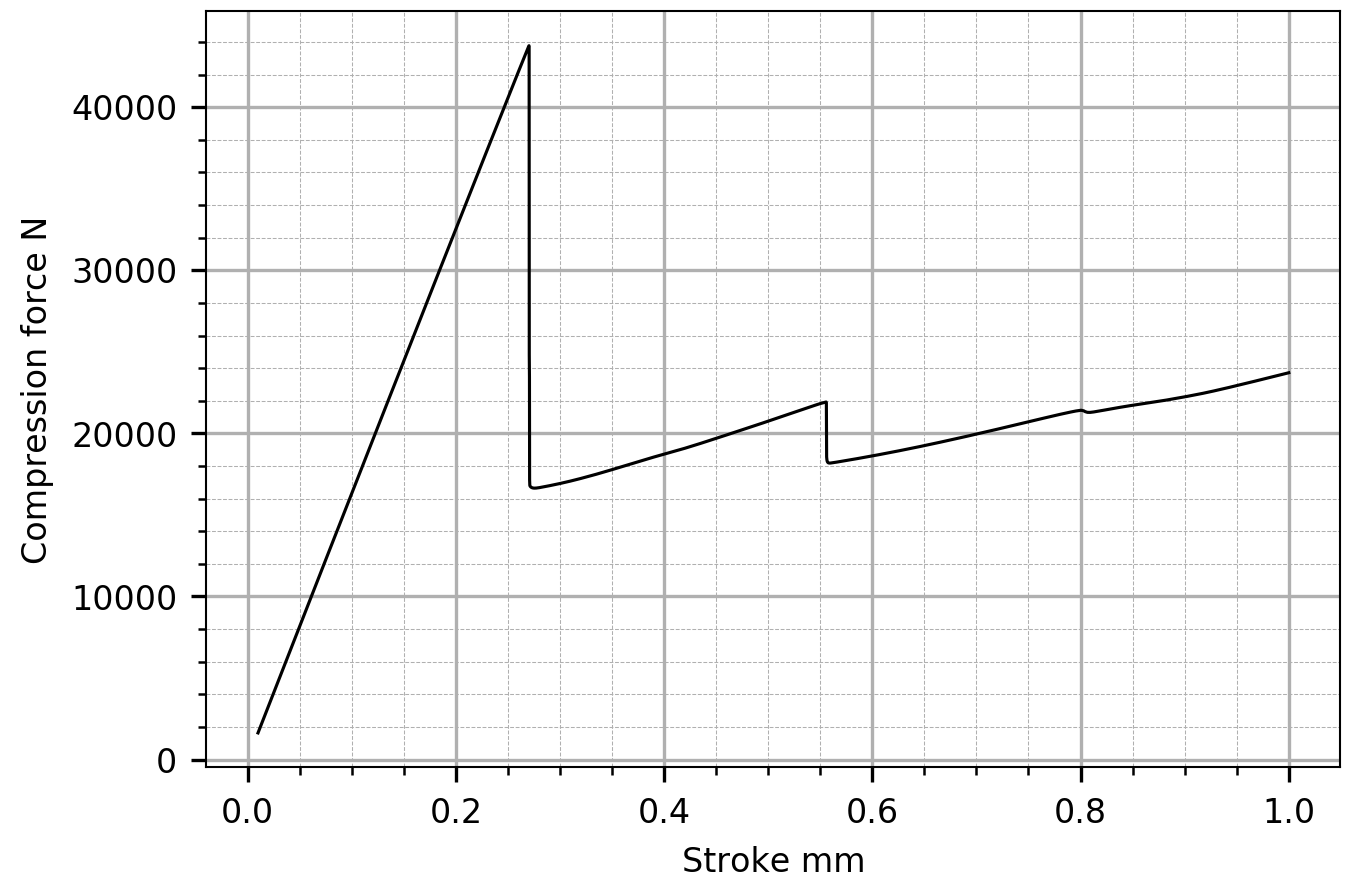
The following data extraction and data manipulation modules are available:
Model
: The Simples base class opens a model database and loads all data except solutions. A wide range of functions allow for accessing and processing of data.NodeField
: Loads and accesses node (DOF) fields.ElementField
: Loads and accesses node (DOF) fields.SamplingPointField
: Loads and accesses Sampling Point Fields, i.e. fields defined element wise, at specific sampling (integration) points. Typically, strains, stresses, or failure criteria are stored in Sampling Point Fields.BeamStressField
: A class for computing beam element stresses. Beam stresses are not contained in Sampling Point Fields, because beam stresses are not computed by B2000++: B2000++ only computes beam section forces and moments.BeamCrossSection
: The beam cross section class for computing and viewing beam cross sections, specifically thin sections and a range of predefined section types.Data Formatters: Extracts database objects, such as fields, and formats them.
Graphs: A basic graph plotting class that makes use of Matplotlib.
Utility modules and functions.